Title:Dac Using PIC18F4520
Program:
//Author : Palak Patel
//Contact No:9173211683
//Title:dac
//Platform: PIC18f4520
//Software:MPLAB
#include<p18f4520.h>
#pragma config OSC = INTIO67
#pragma config FCMEN = OFF
#pragma config IESO = OFF
#pragma config PWRT = OFF
#pragma config BOREN = OFF
#pragma config WDT = OFF
#pragma config MCLRE = ON
#pragma config PBADEN = OFF
#pragma config STVREN = OFF
#pragma config LVP = OFF
#define sw0 PORTCbits.RC0
#define sw1 PORTCbits.RC1
void cmd(unsigned char);
void dat(unsigned char);
void Delay_ms(unsigned char);
void delay();
#define dac PORTD
void delay()
{
unsigned int p;
for(p=0;p<1000;p++);
}
void main()
{
unsigned int value,value1,i,Temp,x[25],j,a,b,c,d,p,e,f,g,h;
unsigned char name[]="Voltage Difference=";
TRISB=0x00;
TRISD=0x00;
TRISAbits.TRISA0=1;
TRISAbits.TRISA2=1;
TRISCbits.TRISC0=1;
TRISCbits.TRISC2=1;
ADCON=0x0F;
cmd(0x01); //Clear Lcd
cmd(0x02); //4 Bit Specification
cmd(0x28); //4 Bit specification
cmd(0x0c);
cmd(0x80); //Cursor starting point
cmd(0X06);
i=0;
while(name[i]!='\0')
{
dat(name[i]);
i++;
}
cmd(0xc0);
i=0x00;
dac=0x00;
while(1)
{
if(sw0 == 0)
{
delay();
delay();
if(sw0 == 0)
{
i=i+1;
dac=i;
delay();
}
}
if(sw1 == 0)
{
delay();
delay();
if(sw1 == 0)
{
i=i-1;
dac=i;
delay();
}
}
dac=i;
delay();
}
}
Simulation:
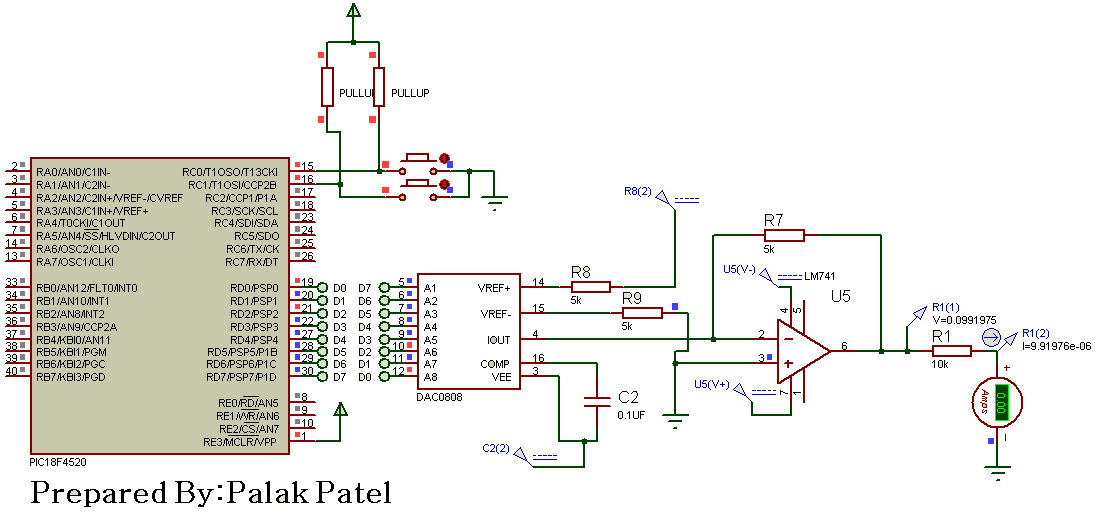
Program:
//Author : Palak Patel
//Contact No:9173211683
//Title:dac
//Platform: PIC18f4520
//Software:MPLAB
#include<p18f4520.h>
#pragma config OSC = INTIO67
#pragma config FCMEN = OFF
#pragma config IESO = OFF
#pragma config PWRT = OFF
#pragma config BOREN = OFF
#pragma config WDT = OFF
#pragma config MCLRE = ON
#pragma config PBADEN = OFF
#pragma config STVREN = OFF
#pragma config LVP = OFF
#define sw0 PORTCbits.RC0
#define sw1 PORTCbits.RC1
void cmd(unsigned char);
void dat(unsigned char);
void Delay_ms(unsigned char);
void delay();
#define dac PORTD
void delay()
{
unsigned int p;
for(p=0;p<1000;p++);
}
void main()
{
unsigned int value,value1,i,Temp,x[25],j,a,b,c,d,p,e,f,g,h;
unsigned char name[]="Voltage Difference=";
TRISB=0x00;
TRISD=0x00;
TRISAbits.TRISA0=1;
TRISAbits.TRISA2=1;
TRISCbits.TRISC0=1;
TRISCbits.TRISC2=1;
ADCON=0x0F;
cmd(0x01); //Clear Lcd
cmd(0x02); //4 Bit Specification
cmd(0x28); //4 Bit specification
cmd(0x0c);
cmd(0x80); //Cursor starting point
cmd(0X06);
i=0;
while(name[i]!='\0')
{
dat(name[i]);
i++;
}
cmd(0xc0);
i=0x00;
dac=0x00;
while(1)
{
if(sw0 == 0)
{
delay();
delay();
if(sw0 == 0)
{
i=i+1;
dac=i;
delay();
}
}
if(sw1 == 0)
{
delay();
delay();
if(sw1 == 0)
{
i=i-1;
dac=i;
delay();
}
}
dac=i;
delay();
}
}
Simulation:
For Basic Electronics Kindaly follow this Link: